Node.js is a tool that is very familiar to web developers. But have you ever delved into how this powerful tool works, especially what role the event loop plays? The event loop is the core element that handles all asynchronous operations in Node.js, and understanding it properly is key to optimizing Node.js performance. In this article, we’ll explore the inner workings of the event loop and how this technology impacts web development.
The Basics of the Event Loop
When a Node.js application runs, the event loop continuously operates to manage all asynchronous tasks. The event loop runs on a single main thread, but this main thread allows other asynchronous tasks to be processed on separate threads. For example, tasks like file reading and network requests are executed asynchronously, and when completed, the results are passed back to the event loop via callback functions.

Collaboration Between the Main Thread and Worker Threads
In addition to the event loop, Node.js uses a library called Libuv to manage multiple worker threads. These worker threads handle heavy tasks like file reading, network requests, and encryption. The event loop receives the results and calls the necessary callback functions. An important point here is that the main thread can keep running without waiting for asynchronous tasks to complete, allowing it to handle other operations.

Asynchronous Processing in JavaScript
Many people simply understand asynchronous processing in Node.js through JavaScript’s promises or the `async/await` keywords. However, in reality, all these operations are managed by the event loop, and various queues are used in this process. For example, methods like `process.nextTick()` register callbacks in a special queue called the nextTick queue to execute them as soon as possible. Thanks to these mechanisms, Node.js can process asynchronous tasks very efficiently.
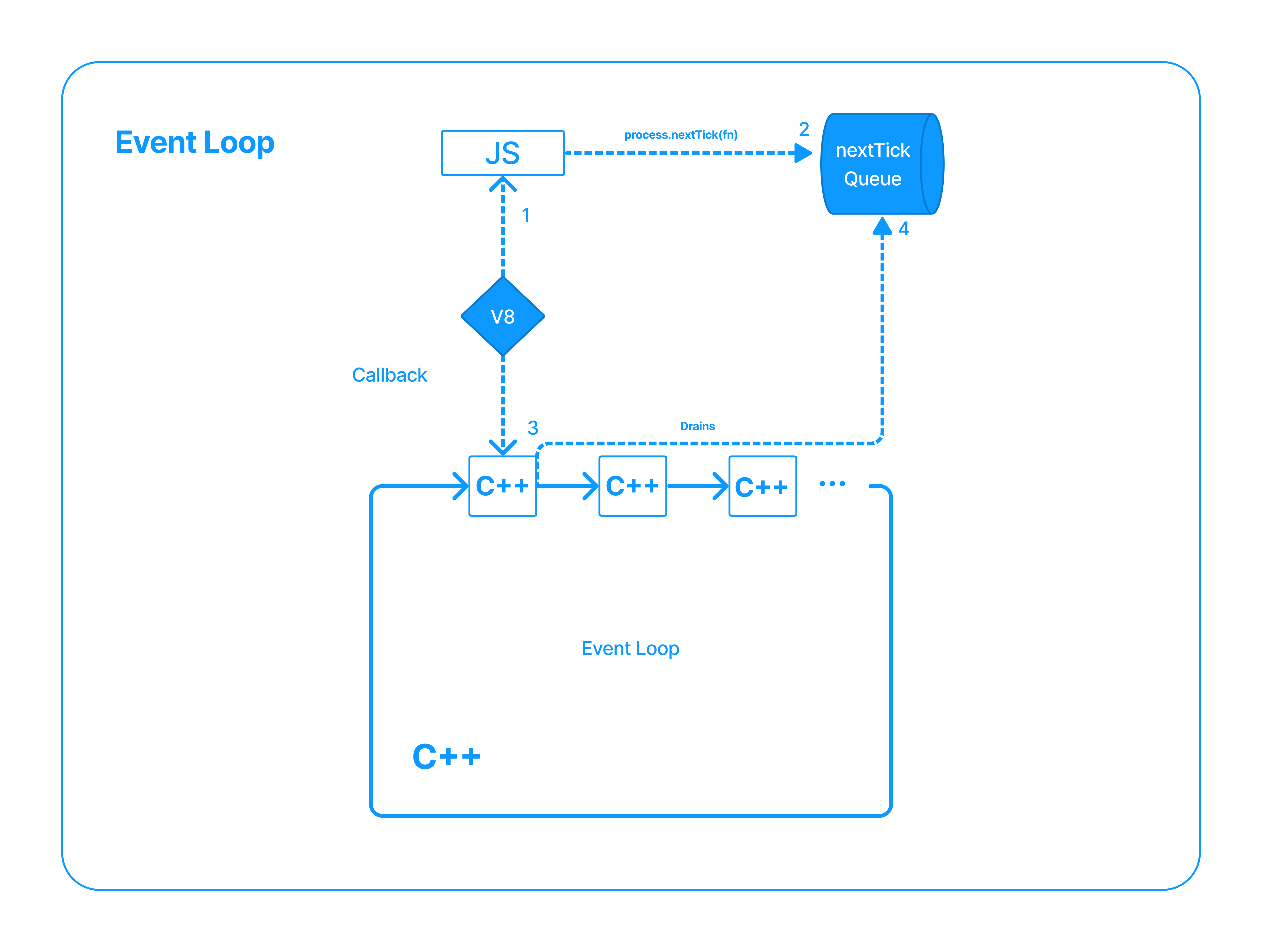
Understanding the Event Loop Phases
The event loop goes through several phases in each iteration. Each phase is used to process specific tasks and is executed in the following order:
- Timers Phase: Callbacks scheduled by `setTimeout()` and `setInterval()` are executed in this phase.
- I/O Callbacks Phase: Asynchronous I/O task callbacks are executed in this phase.
- Event Queue Processing Phase: Callbacks like those from `process.nextTick()` are processed here.
- Phases Repeated: After each phase is completed, the loop repeats the process.
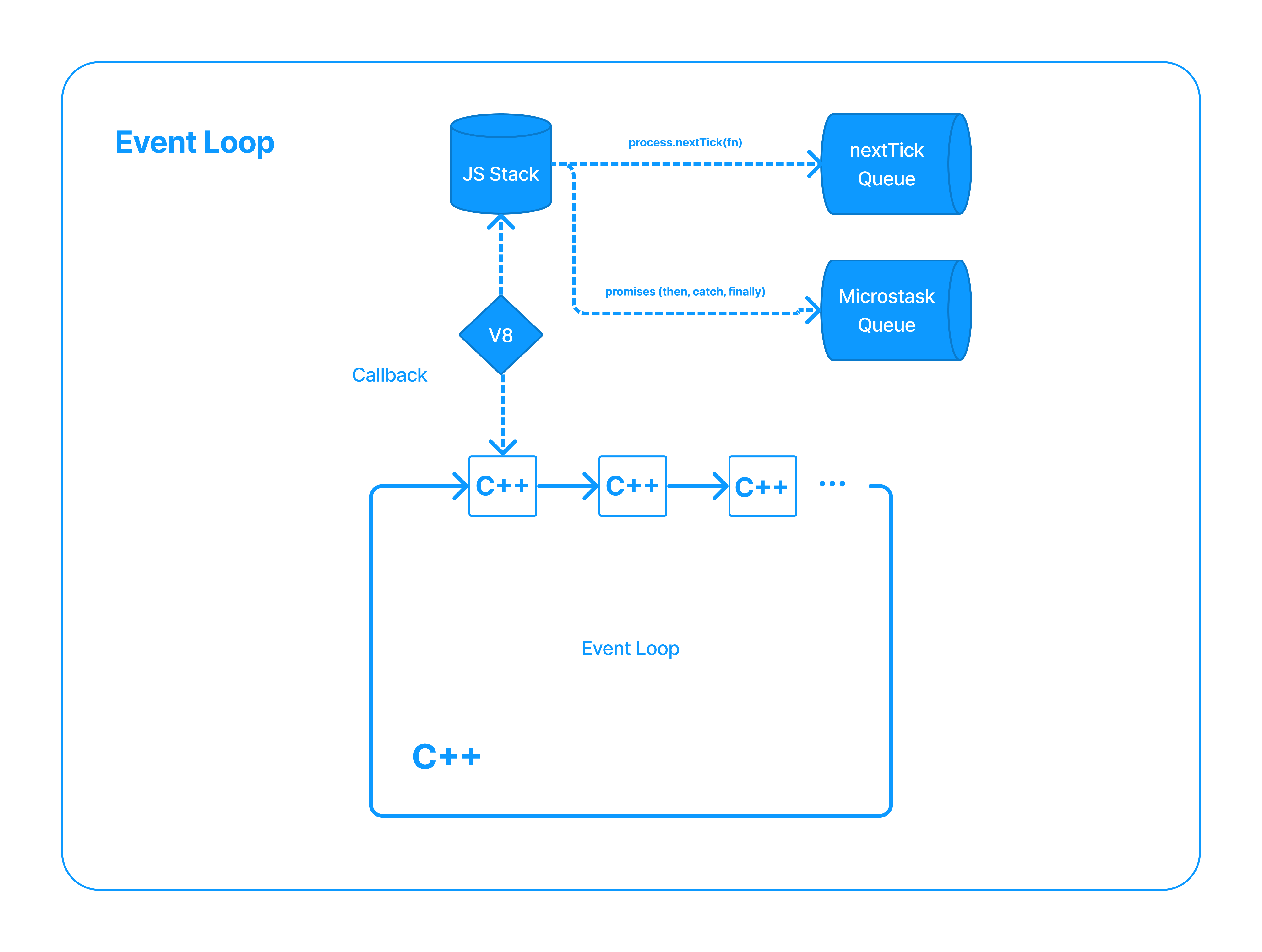
Thanks to the continuous execution of the event loop during this process, Node.js applications can efficiently handle multiple asynchronous tasks.
The Role of Streams and Event Emitters
In Node.js, streams and event emitters help make asynchronous tasks easier to handle. For example, when reading a file or processing network requests, streams allow data to be handled in small chunks. This reduces memory usage and is very useful for processing larger data.
Event emitters play a crucial role in Node.js. Objects like streams use event emitters to handle specific events as they occur. For instance, while reading a file, the `data` event allows data to be processed whenever it is received.
Understanding the event loop is essential when developing web applications. For example, properly utilizing the asynchronous processing method of the event loop can maximize concurrent processing performance. This is especially impactful in large-scale applications that need to handle a lot of traffic.

Conclusion
The Node.js event loop is not just a technical concept but a crucial factor that significantly impacts the performance of practical applications. By understanding and properly utilizing it, you can develop faster and more efficient web applications. For those looking to deepen their understanding of Node.js, we encourage you to start exploring various internal mechanisms of Node.js, beginning with the event loop.
Reference: ThisDot Media, “Deep Dive into Node.js with James Snell”