This article explains how to use the Naver Shopping API to fetch product lists from a specific shop in a React application, step by step. By utilizing the Naver Shopping API, you can easily access various shopping-related data, enabling efficient shopping mall data management.
To use the API, you need to obtain an API key from the Naver Developer Center. Detailed usage and examples of each API can be found in the Naver Developer Center official documentation.
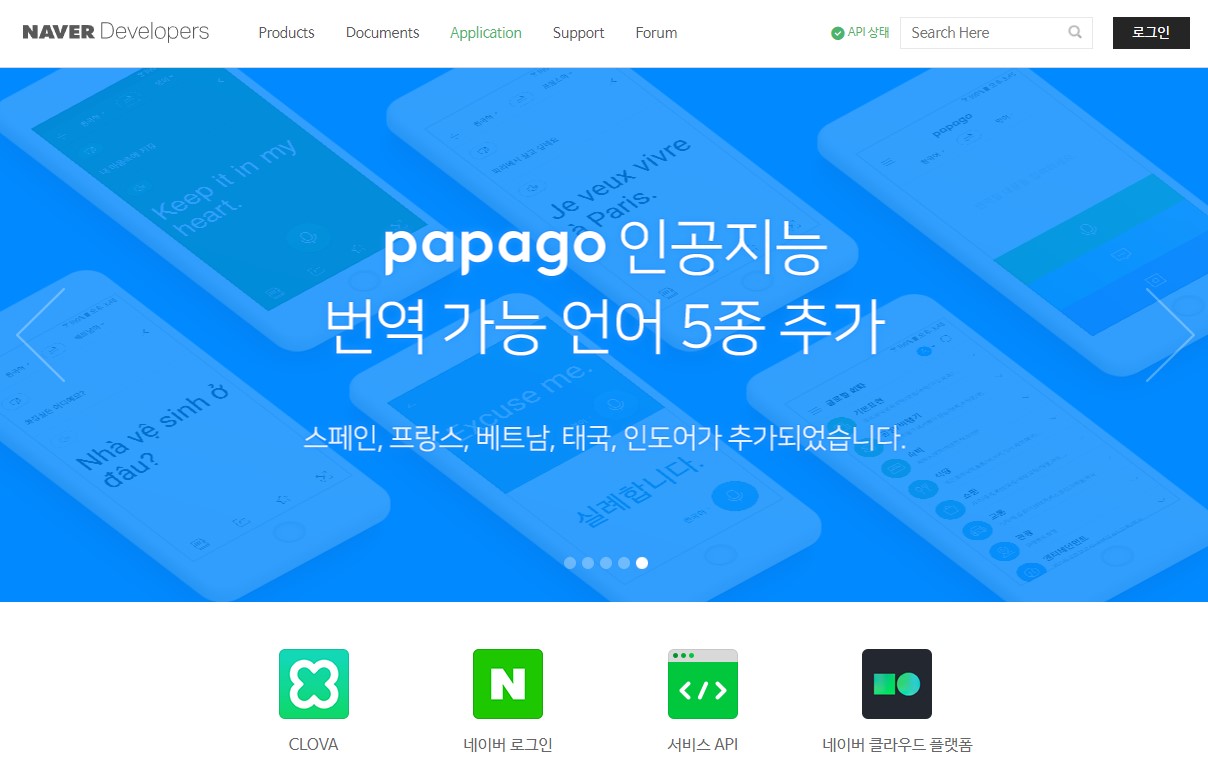
Overview of Naver Shopping API
The Naver Shopping API provides various features for searching and retrieving product information. The main APIs are as follows:
- Product Search API: Search for products using specific keywords and retrieve information such as product name, image, price, and seller details.
- Product Detail API: Retrieve detailed information about a specific product.
- Category Information API: Get the category structure of Naver Shopping.
- Shop-specific Product Search API: Search for products sold by a specific shop.
- Price Comparison API: Compare prices of the same product sold by different shops.
Setting Up React Project and Integrating API
1. Setting Up React Project
First, use Create React App to set up a new React project. Run the following commands in the terminal:
npx create-react-app naver-shopping
cd naver-shopping
npm install axios
2. Storing API Key in .env File
Create a `.env` file in the project root directory to store the Naver API key:
REACT_APP_NAVER_CLIENT_ID=YOUR_CLIENT_ID
REACT_APP_NAVER_CLIENT_SECRET=YOUR_CLIENT_SECRET
3. Writing React Component
Open the `App.js` file and write the following code. This code uses axios to call the Naver API and fetches the product list from a specific shop, then renders it on the screen.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
import './App.css';
function App() {
const [products, setProducts] = useState([]);
const [error, setError] = useState(null);
useEffect(() => {
const fetchProducts = async () => {
try {
const response = await axios.get('https://openapi.naver.com/v1/search/shop.json', {
params: {
query: 'specific shop name', // Enter the specific shop name here.
display: 10,
start: 1,
sort: 'sim'
},
headers: {
'X-Naver-Client-Id': process.env.REACT_APP_NAVER_CLIENT_ID,
'X-Naver-Client-Secret': process.env.REACT_APP_NAVER_CLIENT_SECRET
}
});
setProducts(response.data.items);
} catch (err) {
setError(err);
}
};
fetchProducts();
}, []);
return (
<div className="App">
<h1>Product List</h1>
{error && <p>Error fetching products: {error.message}</p>}
<ul>
{products.map(product => (
<li key={product.link}>
<a href={product.link} target="_blank" rel="noopener noreferrer">
<img src={product.image} alt={product.title} />
<p>{product.title}</p>
<p>{product.lprice} 원</p>
</a>
</li>
))}
</ul>
</div>
);
}
export default App;
4. Adding Styles
Add simple styles to the `App.css` file to improve the UI:
.App {
font-family: Arial, sans-serif;
text-align: center;
}
ul {
list-style: none;
padding: 0;
}
li {
margin: 10px 0;
}
img {
width: 100px;
height: 100px;
}
a {
text-decoration: none;
color: black;
}
5. Running the Application
Run the following command in the project root directory to start the application:
npm start
You can check the product list from the specific shop fetched using the Naver Shopping API by visiting `http://localhost:3000` in your browser.
Conclusion
In this article, we looked at how to use the Naver Shopping API to fetch product lists from a specific shop in a React application. Using the API allows you to easily retrieve and utilize various product information, which can greatly help in managing and operating a shopping mall. By understanding the basics of using the API and integrating it with React through this example, you can implement more complex functionalities in your applications.