Easily Understanding the React Compiler’s Type System
For those encountering the React compiler’s type system for the first time, it can seem quite challenging. However, by gradually unraveling its complexity through this article, it will become much easier to understand.
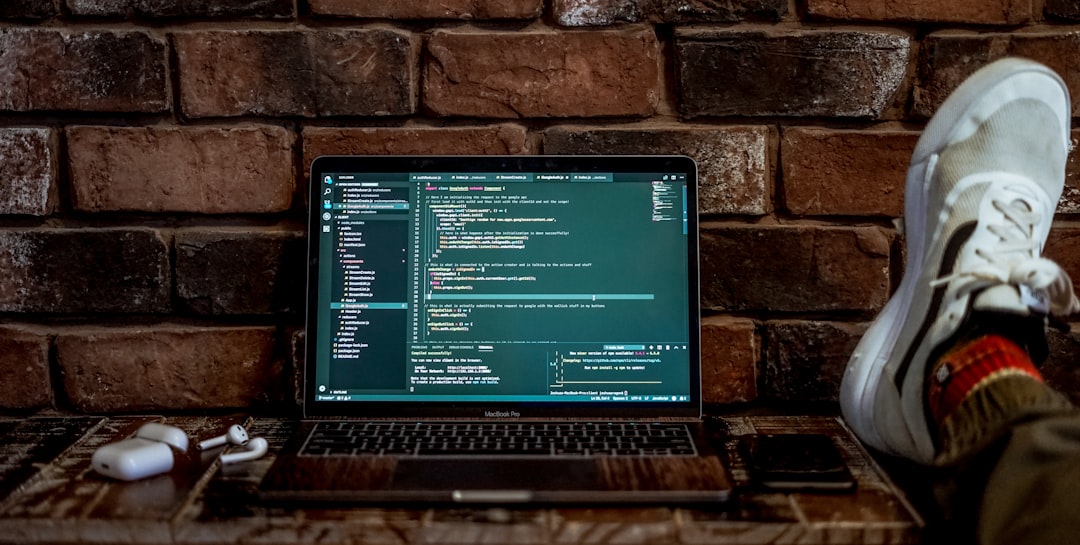
React Compiler’s Type System
The type system of the React compiler can feel complex to many developers. However, understanding its basics can provide clearer insights into how the React compiler operates.
1. Property Memoization
In React, a component wrapped with `React.memo` only re-renders when its properties change. This significantly aids in performance optimization.
const Greeting = memo(function Greeting({ user }) {
return (
<h1>
Hello, {user.firstName} {user.lastName}!
</h1>
);
});
In the code above, the `Greeting` component only re-renders when the `user` property changes.
This approach is very useful for optimizing performance. However, not all cases of property memoization are valid.
2. Importance of Type Inference
Type inference is the process by which the compiler automatically determines the types in the code. The React compiler uses this to enhance code stability.
function Price({ items, state }) {
const subTotal = calculateSubTotal(items);
const tax = calculateTax(subTotal, state);
const total = subTotal + tax;
return <Text text={total} />;
}
In this example, the `Price` component calculates the total based on `items` and `state`. The React compiler infers this value to avoid unnecessary memoization.
3. Creating Type Equations
Type equations are used to determine the types in the code. They are expressed as statements that show the equivalence between type variables and types.
const total = subTotal + tax;
In this code, `subTotal` and `tax` are considered primitive types. This plays a crucial role in the compiler’s type inference process.
4. Unification Process of Type Inference
The unification process is where the type equations are solved. This allows the compiler to accurately determine the types in the code.
const subTotal = calculateSubTotal(items);
The compiler infers the return type of the `calculateSubTotal` function to determine the type of `subTotal`. This process ensures accurate type inference in the code.
5. Necessity of Property Memoization
The React compiler can infer that all values are primitive types. This helps prevent unnecessary memoization, saving bundle size and memory.
function Price(t0) {
const $ = useMemoCache(2);
const { items, state } = t0;
const subTotal = calculateSubTotal(items);
const tax = calculateTax(subTotal, state);
const total = subTotal + tax;
let t1;
if ($[0] !== total) {
t1 = <Text text={total} />;
$[0] = total;
$[1] = t1;
} else {
t1 = $[1];
}
return t1;
}
This code greatly helps in saving memory and bundle size.
Conclusion
The type system of the React compiler plays a crucial role in optimizing code stability and performance. It allows developers to maintain better code quality.
By understanding the React compiler’s type system, you can write more efficient and stable code. Try leveraging the React compiler’s type system yourself!
Reference: Recompiled.dev, “Type System”