JavaScript’s Set object provides a collection of unique values, making it a useful tool for eliminating duplicate items. With the recent Firefox 127 update, new Set methods have been introduced, and now these methods can be used across various browsers. These new methods help developers manage Set objects more easily and efficiently. Let’s take a closer look at the functionalities and usage of these newly added methods.
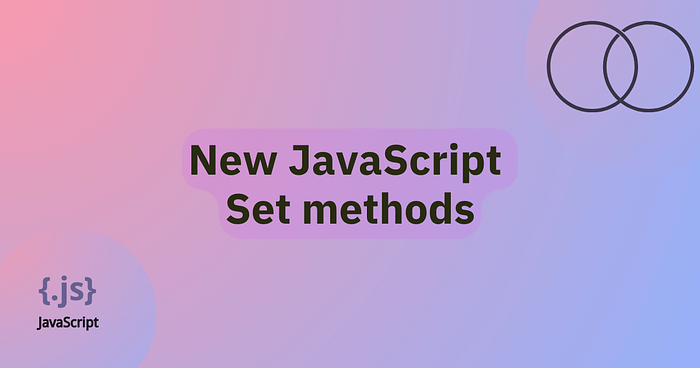
Overview of New Set Methods
The new methods added to the Set object are as follows:
- intersection()
- union()
- difference()
- symmetricDifference()
- isSubsetOf()
- isSupersetOf()
- isDisjointFrom()
These methods make the Set object more flexible and powerful. Let’s briefly go over what each method does.
1. intersection()
The `intersection()` method returns a new Set containing the common elements of two Sets.
const setA = new Set([1, 2, 3]);
const setB = new Set([2, 3, 4]);
const intersectionSet = setA.intersection(setB);
console.log(intersectionSet); // Set { 2, 3 }
2. union()
The `union()` method returns a new Set containing all elements from two Sets.
const setA = new Set([1, 2, 3]);
const setB = new Set([2, 3, 4]);
const unionSet = setA.union(setB);
console.log(unionSet); // Set { 1, 2, 3, 4 }
3. difference()
The `difference()` method returns a new Set containing elements present in the first Set but not in the second Set.
const setA = new Set([1, 2, 3]);
const setB = new Set([2, 3, 4]);
const differenceSet = setA.difference(setB);
console.log(differenceSet); // Set { 1 }
4. symmetricDifference()
The `symmetricDifference()` method returns a new Set containing elements that are in either of the Sets but not in both.
const setA = new Set([1, 2, 3]);
const setB = new Set([2, 3, 4]);
const symmetricDifferenceSet = setA.symmetricDifference(setB);
console.log(symmetricDifferenceSet); // Set { 1, 4 }
5. isSubsetOf()
The `isSubsetOf()` method returns a boolean value indicating whether all elements of the current Set are contained within another Set.
const setA = new Set([1, 2]);
const setB = new Set([1, 2, 3]);
console.log(setA.isSubsetOf(setB)); // true
6. isSupersetOf()
The `isSupersetOf()` method returns a boolean value indicating whether the current Set contains all elements of another Set.
const setA = new Set([1, 2, 3]);
const setB = new Set([1, 2]);
console.log(setA.isSupersetOf(setB)); // true
7. isDisjointFrom()
The `isDisjointFrom()` method returns a boolean value indicating whether two Sets share no common elements.
const setA = new Set([1, 2]);
const setB = new Set([3, 4]);
console.log(setA.isDisjointFrom(setB)); // true
Importance of New Methods
These methods provide significant convenience to developers. For example, they make it easy to remove duplicate elements and clearly understand the relationships between two Sets. This is particularly useful when handling large datasets or complex data structures.
// Example: Creating a unique user list
const onlineUsers = new Set(['Alice', 'Bob', 'Charlie']);
const offlineUsers = new Set(['Bob', 'Charlie', 'David']);
// All users who are either online or offline
const allUsers = onlineUsers.union(offlineUsers);
console.log(allUsers); // Set { 'Alice', 'Bob', 'Charlie', 'David' }
// Users who are both online and offline
const commonUsers = onlineUsers.intersection(offlineUsers);
console.log(commonUsers); // Set { 'Bob', 'Charlie' }
// Users who are only online
const onlyOnlineUsers = onlineUsers.difference(offlineUsers);
console.log(onlyOnlineUsers); // Set { 'Alice' }
// Users who are either online or offline, but not both
const uniqueUsers = onlineUsers.symmetricDifference(offlineUsers);
console.log(uniqueUsers); // Set { 'Alice', 'David' }
In this way, the Set object and its new methods become powerful tools for effectively managing and manipulating data. They allow you to solve complex problems in a simple and clear manner.
Conclusion
The new methods in JavaScript’s Set object offer numerous benefits to developers. These methods enable more efficient data management and manipulation, and can be useful in a variety of situations. Learn and utilize these new methods to make your development tasks simpler and more effective!
Reference: MDN Web Docs, “JavaScript Set Methods”