The problem every developer can relate to: repeatedly setting up ESLint and Prettier at the start of each project. This task is tedious, time-consuming, and maintaining code consistency is difficult if team members have different configurations. To solve this issue, we’ll introduce a method for creating a shared config for your team. This article will cover how to effectively set up ESLint and Prettier, and how to enhance overall team productivity.
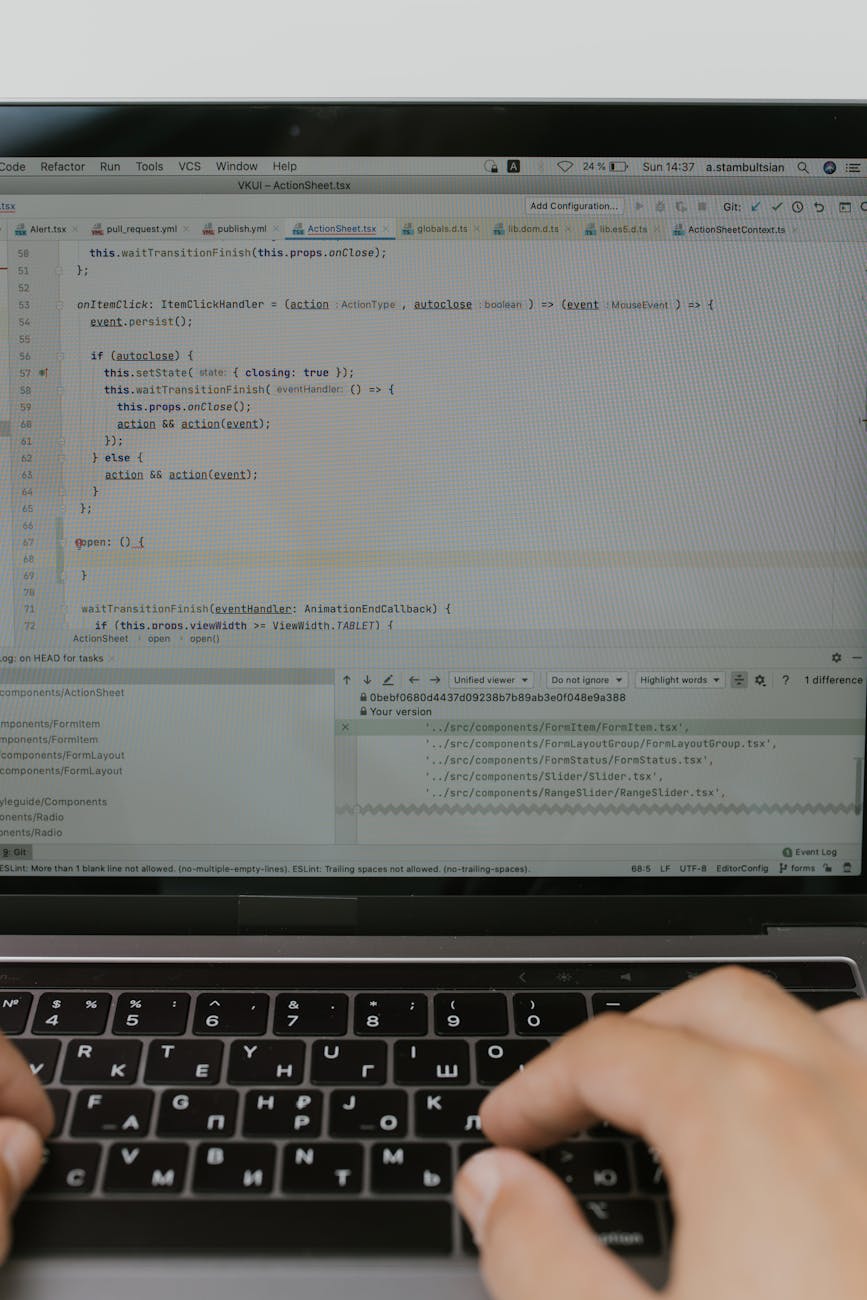
The Need for Shared Configs
ESLint and Prettier are essential tools for maintaining code quality and consistent formatting in JavaScript or TypeScript projects. However, repeating ESLint/Prettier settings for every new project is cumbersome. Using a shared config can address these issues.@note
Advantages of ESLint and Prettier
1. ESLint
ESLint helps quickly identify and fix potential issues in your code.@note For instance, it warns about unused variables during declaration. This improves code readability and significantly reduces bugs.
2. Prettier
Prettier automatically formats your code. With this tool, developers can focus solely on writing code without worrying about formatting.@note Consistent code style maximizes team collaboration efficiency.
Setting Up Shared Configs
1. ESLint Shared Config
Let’s start with setting up an ESLint shared config. Many front-end developers use the Airbnb eslint-config-airbnb package as a standard configuration.@note However, this setup can be overly strict, disrupting workflow. As an alternative, you can choose Microsoft’s @rushstack/eslint-config.
{
"name": "@org/eslint-config",
"main": "index.js",
"version": "1.0.0",
"dependencies": {
"@rushstack/eslint-config": "3.6.8",
"@rushstack/eslint-patch": "1.10.1",
"eslint-plugin-react": "7.34.1",
"eslint-plugin-react-hooks": "4.6.0"
},
"peerDependencies": {
"eslint": ">= 8",
"typescript": ">= 5"
}
}
2. Prettier Shared Config
Next, let’s set up a Prettier shared config. Here is a commonly used Prettier configuration.
{
"printWidth": 100,
"tabWidth": 2,
"semi": true,
"singleQuote": true,
"bracketSpacing": true,
"arrowParens": "always",
"useTabs": false
}
Applying ESLint/Prettier to a React Project
Create a Vite-based React project and apply the shared configs. In the project folder, create a `.eslintrc.cjs` file and configure it as follows.
require("@org/eslint-config/patch");
module.exports = {
env: { browser: true, es2020: true },
extends: [
"@org/eslint-config",
"@org/eslint-config/mixins/react"
],
settings: {
react: {
version: "18.2"
}
},
parserOptions: {
project: true,
tsconfigRootDir: __dirname
}
};
Recommended Rules and Plugins
1. Naming Convention Rules
Using the `@typescript-eslint/naming-convention` rule from the @typescript-eslint/eslint-plugin, you can define conventions for naming. For instance, you can set it so that interface names do not start with `I`.
{
"rules": {
"@typescript-eslint/naming-convention": [
"warn",
{ "selector": "variable", "format": ["camelCase", "PascalCase", "UPPER_CASE"] },
{ "selector": "function", "format": ["camelCase", "PascalCase"] },
{ "selector": "typeLike", "format": ["PascalCase"] },
{ "selector": "interface", "format": ["PascalCase"], "custom": { "regex": "^I[A-Z]", "match": false } },
{ "selector": "typeAlias", "format": ["PascalCase"], "custom": { "regex": "^T[A-Z]", "match": false } }
]
}
}
2. Enforcing Absolute Import Paths
You can set a rule to enforce using absolute import paths.
{
"rules": {
"no-relative-import-paths/no-relative-import-paths": [
"warn",
{ "allowSameFolder": true, "rootDir": "src", "prefix": "@" }
]
}
}
3. Enforcing Destructuring
You can set a rule to enforce destructuring.
{
"prefer-destructuring": [
"error",
{
"VariableDeclarator": {
"array": false,
"object": true
},
"AssignmentExpression": {
"array": false,
"object": false
}
}
]
}
4. Auto-Sorting Tailwind CSS Classes
Using Prettier’s prettier-plugin-tailwindcss, you can automatically sort Tailwind CSS class names.
{
plugins: ['prettier-plugin-tailwindcss']
}
Conclusion
By implementing shared configs, you can boost your team’s productivity and maintain code consistency. ESLint and Prettier setups greatly aid in smooth collaboration and code quality improvement among team members. Adopting shared config packages can create a better development environment, so consider implementing them to enhance your team’s productivity!