React developers are likely familiar with the nuisance of memory leak issues, especially when using closures and memoization hooks like `useCallback` or `useEffect`. This article explores how the new React compiler can mitigate these problems and the areas where it still falls short.
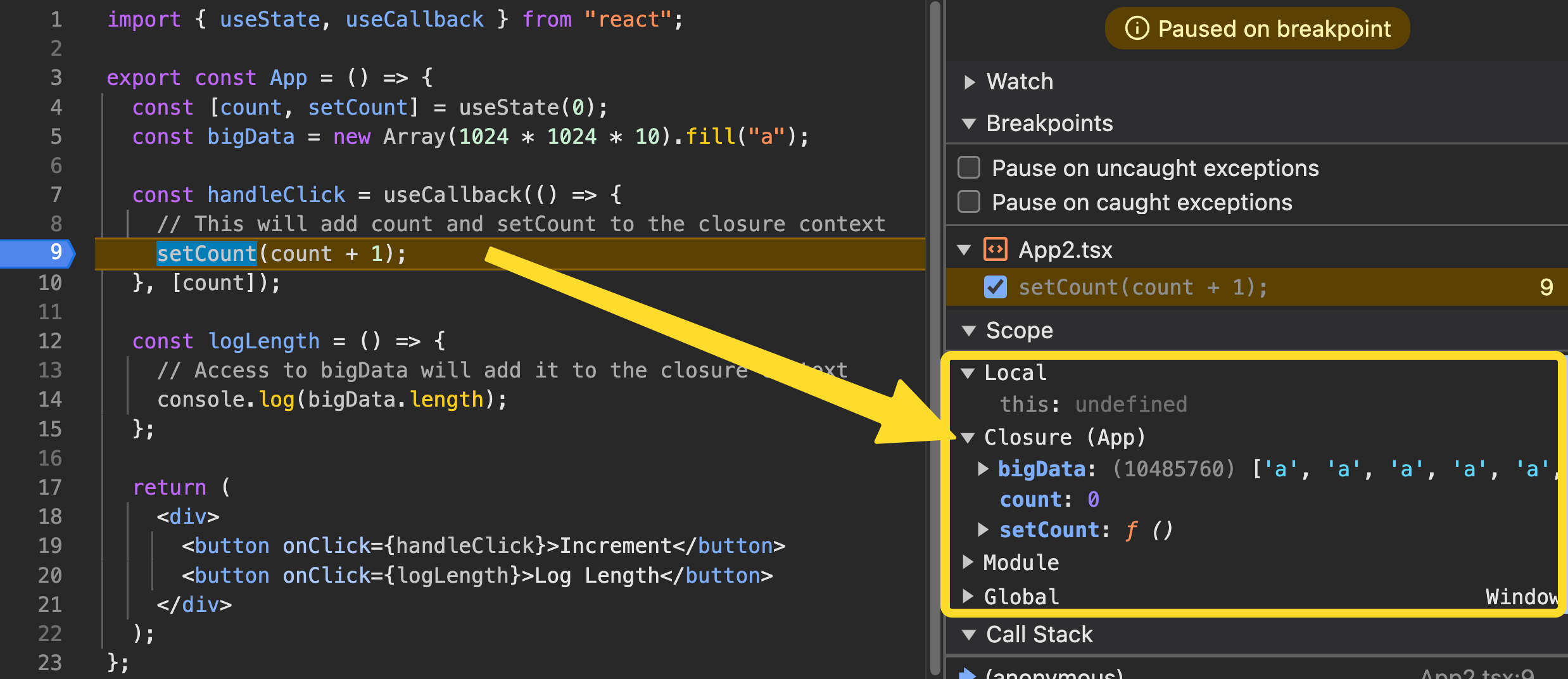
The Limitations of the React Compiler: It Can’t Prevent All Memory Leaks
If you were expecting the new React compiler to completely solve memory leak issues, you might be slightly disappointed. [While the React compiler can prevent memory leaks caused by closures under certain conditions, it doesn’t apply universally.] The React team explains that the compiler can cache certain memoized values to prevent closure-related memory leaks, but leaks can still occur depending on the values referenced by closures.
For example, in the code below, every time the state is changed in the memoized `useCallback` function, a new instance of `BigObject` is created, which isn’t collected by the garbage collector.
import { useState, useCallback } from 'react';
class BigObject {
public readonly data = new Uint8Array(1024 * 1024 * 10);
}
export const App = () => {
const [countA, setCountA] = useState(0);
const [countB, setCountB] = useState(0);
const bigData = new BigObject();
const handleClickA = useCallback(() => {
setCountA(countA + 1);
}, [countA]);
const handleClickB = useCallback(() => {
setCountB(countB + 1);
}, [countB]);
const handleClickBoth = () => {
handleClickA();
handleClickB();
console.log(bigData.data.length);
};
return (
<div>
<button onClick={handleClickA}>Increment A</button>
<button onClick={handleClickB}>Increment B</button>
<button onClick={handleClickBoth}>Increment Both</button>
<p>A: {countA}, B: {countB}</p>
</div>
);
};
When this code runs in the React compiler, you’ll notice in the memory snapshot that instances of `BigObject` keep being allocated. This occurs because new instances are created with each state change, leading to a memory leak.
Approaches to Resolving the Issue
One way to address this issue is to bypass closures entirely. For instance, by using the `bind` method to pass values directly to a function, you avoid reliance on the shared context object across closures. This approach helps prevent memory leaks.
Here is an example using `bind`:
import { useState } from 'react';
class BigObject {
constructor(public state: string) {}
public readonly data = new Uint8Array(1024 * 1024 * 10);
}
function bindNull<U extends unknown[]>(f: (args: U) => void, x: U): () => void {
return f.bind(null, x);
}
export const App = () => {
const [countA, setCountA] = useState(0);
const [countB, setCountB] = useState(0);
const bigData = new BigObject(`${countA}/${countB}`);
const handleClickA = bindNull(([count, setCount]) => {
setCount(count + 1);
}, [countA, setCountA] as const);
const handleClickB = bindNull(([count, setCount]) => {
setCount(count + 1);
}, [countB, setCountB] as const);
const handleClickBoth = bindNull(([countA, setCountA, countB, setCountB]) => {
setCountA(countA + 1);
setCountB(countB + 1);
console.log(bigData.data.length);
}, [countA, setCountA, countB, setCountB] as const);
return (
<div>
<button onClick={handleClickA}>Increment A</button>
<button onClick={handleClickB}>Increment B</button>
<button onClick={handleClickBoth}>Increment Both</button>
<p>A: {countA}, B: {countB}</p>
</div>
);
};
This code operates as expected in the React compiler without causing memory leaks.
Conclusion
While the React compiler can enhance performance through code optimization, it can’t prevent all memory leaks. [When working with React, be cautious with closures and memoization, and follow best practices to prevent memory leaks.] Writing small, pure components and using memory profilers to identify issues are crucial steps.
Understanding and addressing this issue will significantly improve your React development skills. Take a moment today to inspect your code for potential memory leaks!
Reference: Schiener.io, “Sneaky React Memory Leaks: How the React compiler won’t save you”